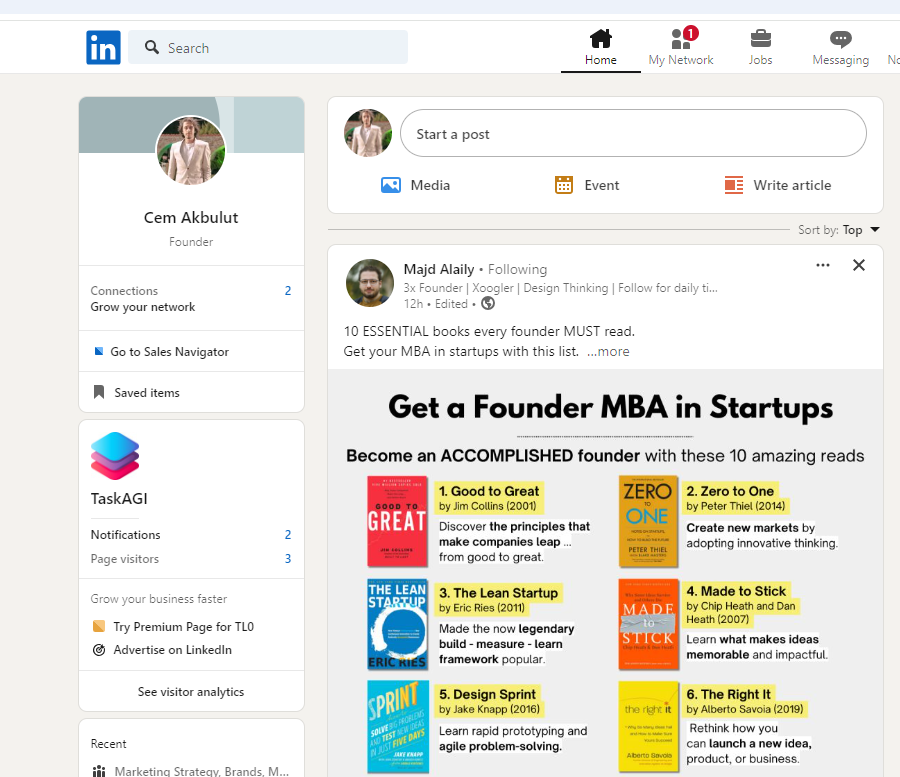
Gathering data from LinkedIn posts can provide valuable insights for various purposes such as professional analysis, industry trend tracking, or competitor benchmarking. However, LinkedIn’s dynamic structure and strict regulations make direct scraping a complex endeavor. Fortunately, TaskAGI’s LinkedIn Scraper API offers a streamlined solution to extract relevant data effortlessly while ensuring compliance with LinkedIn’s guidelines.
In this guide, we will cover how to scrape LinkedIn posts using TaskAGI’s API with Python. TaskAGI offers three distinct endpoints, allowing you to retrieve posts by specific post ID, by URL, or via a user’s profile.
Getting Started
To begin, make sure you have access to TaskAGI’s LinkedIn Scraper API. You can explore more details about the API at LinkedIn Scraper or through RapidAPI.
Once you have registered and received your API key, you are ready to integrate it into your Python script.
Prerequisites
To use the LinkedIn Scraper API, you will need the following:
- Python 3.6 or higher
- The
requests
library to handle HTTP requests
You can install the requests
library by running:
pip install requests
Endpoints Overview
TaskAGI provides three main endpoints for scraping LinkedIn posts:
Endpoint | Description |
---|---|
/posts-by-id | Retrieve a LinkedIn post by its unique ID. |
/posts-by-url | Extract information from a LinkedIn post by URL. |
/posts-by-profile | Get all posts published by a LinkedIn profile. |
Refer to the TaskAGI LinkedIn documentation for more information on how to use each endpoint.
Sample Python Script for Scraping LinkedIn Posts
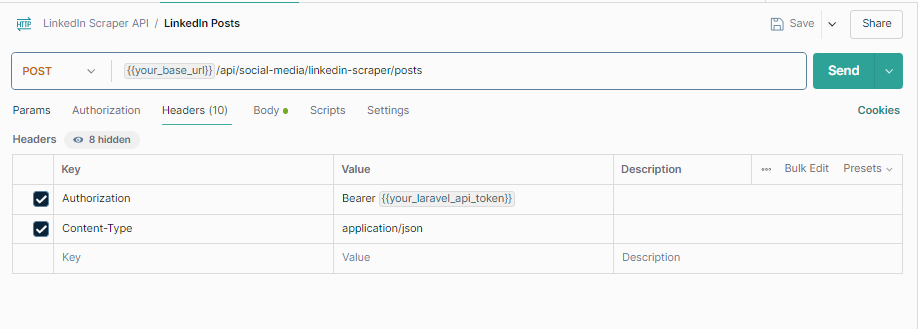
Below is an example of how to use Python to scrape LinkedIn posts using TaskAGI’s LinkedIn Scraper API:
import requests
import json
# API details
api_url = "https://taskagi.net/api/social-media/linkedin-scraper/posts-by-url"
api_key = "YOUR_API_KEY" # Replace with your actual API key
# Parameters for scraping
payload = {
"url": "https://www.linkedin.com/pulse/ab-test-optimisation-earlier-decisions-new-readout-de-b%C3%A9naz%C3%A9"
}
# Headers with API key for authentication
headers = {
"Authorization": f"Bearer {api_key}",
"Content-Type": "application/json"
}
# Function to scrape LinkedIn post
def scrape_linkedin_post():
response = requests.post(api_url, headers=headers, json=payload)
if response.status_code == 200:
data = response.json()
print(json.dumps(data, indent=4)) # Pretty-print the JSON response
else:
print(f"Error: {response.status_code}")
print(response.text)
# Run the function to scrape posts
if __name__ == "__main__":
scrape_linkedin_post()
Explanation of Script
- API URL & Authentication: The API URL is set up for the
/posts-by-url
endpoint. You need to replaceYOUR_API_KEY
with your actual TaskAGI API key for authentication. - Payload: The script includes a payload containing the URL of the LinkedIn post you want to scrape. Modify this to scrape different posts.
- HTTP Headers: The headers include the API key for secure access to the API.
- Request Execution: The
scrape_linkedin_post()
function sends a POST request to the API, and if successful, prints the retrieved data in a formatted JSON structure.
Extended Use Cases
Scraping LinkedIn posts provides powerful insights in various contexts:
- Content Engagement Analysis: Scrape posts to analyze user engagement metrics such as likes and comments. This information is helpful in determining what content resonates most with an audience.
- Competitor Content Tracking: Follow posts from competitors and industry leaders to understand emerging trends and benchmark your performance.
- Lead Generation & Networking: Identify valuable conversations in posts and comments that can lead to potential partnerships or client acquisitions.
- Social Proof and Testimonials: Extract testimonials or positive comments from LinkedIn posts to showcase social proof for your product or service.
- Job Market Trends: Analyze posts by recruiters and companies to track job market trends, in-demand skills, and hiring activity.
Customizing the Script for Enhanced Functionality
TaskAGI’s LinkedIn Scraper API allows you to extract a range of data points, including likes, comments, hashtags, images, and more. You can enhance the Python script to perform additional actions like:
- Save Data to CSV: You can save the scraped post data to a CSV file for further analysis:
import pandas as pd
# Save scraped posts to CSV
def save_to_csv(data):
df = pd.DataFrame([data])
df.to_csv("linkedin_post_data.csv", index=False)
print("Data saved to linkedin_post_data.csv")
- Error Handling: Add error handling to retry requests or log failures:
import time
def scrape_with_retries(retries=3):
for attempt in range(retries):
response = requests.post(api_url, headers=headers, json=payload)
if response.status_code == 200:
return response.json()
else:
print(f"Attempt {attempt + 1} failed: {response.status_code}")
time.sleep(2) # Wait before retrying
return None
- Extracting Specific Information: Use filters to extract only specific fields, such as comments or hashtags, from the scraped data.
Comparison with Competitors’ Features
Many LinkedIn scraping tools in the market, such as PhantomBuster, Apify, and Octoparse, offer similar functionalities but often lack specific flexibility or have limitations like high costs, inconsistent data delivery, or restricted customization options. Here’s how TaskAGI stands out:
- Ease of Use: TaskAGI’s API is beginner-friendly, requiring minimal setup to get started with scraping LinkedIn posts, unlike some other tools that require complex workflows.
- Direct Endpoint Access: With TaskAGI, you get straightforward access to dedicated endpoints such as
/posts-by-id
,/posts-by-url
, and/posts-by-profile
. Other tools may require setting up multiple modules or creating custom workflows to accomplish the same task. - Data Depth: TaskAGI’s API provides a deep dive into LinkedIn post details, including comments, hashtags, engagement metrics, and related media—making it ideal for users interested in comprehensive social media analysis.
- Compliance: TaskAGI emphasizes compliance and offers robust documentation to ensure users understand how to scrape public data in a legal and ethical manner. Competitors often overlook compliance, leaving users vulnerable to potential risks.
- More Data: Along with LinkedIn posts, TaskAGI’s LinkedIn scraper also lets you scrape other data from the platform such as companies, job listings, profiles and more at no extra cost.
Sample Response
An example of a successful response from TaskAGI’s API may look like this:
[
{
"url": "https://www.linkedin.com/pulse/ab-test-optimisation-earlier-decisions-new-readout-de-b%C3%A9naz%C3%A9",
"id": "ab-test-optimisation-earlier-decisions-new-readout-de-b%C3%A9naz%C3%A9",
"user_id": "guillaume-de-benaze-datama",
"title": "A/B TEST OPTIMISATION: EARLIER DECISIONS WITH NEW READOUT METHODS",
"headline": "Back-testing methodologies to run more AB tests in less time on a website, in order to increase final conversion uplift in a limited time range.",
"date_posted": "2020-12-17T08:37:20.000Z",
"num_likes": 25827,
"num_comments": 115,
"images": [
"https://media.licdn.com/dms/image/v2/C4D12AQEwDbpJbHwT1w/article-inline_image-shrink_400_744/0/1608193404663"
],
"embedded_links": [
"https://guillaume-debenaze.medium.com/a-b-test-optimisation-earlier-decisions"
]
}
]
This response includes details like the post URL, post ID, user ID, title, headline, post date, number of likes and comments, images, and embedded links. All of this information can be used to perform detailed social media analysis.
FAQ
1. Can I scrape private LinkedIn posts?
No, TaskAGI’s LinkedIn Scraper API only allows the scraping of public data, ensuring compliance with LinkedIn’s terms of service. Scraping private posts can lead to account suspension and legal consequences.
2. Are there any limitations on the number of requests I can make?
Yes, TaskAGI enforces rate limits to maintain fair usage and API stability. Refer to the API documentation to learn more about the rate limits applicable to your plan.
3. Can I extract media content like images and videos?
Yes, the API provides URLs to images and videos embedded in LinkedIn posts, making it easy to gather multimedia content.
4. What types of posts can I scrape?
You can scrape individual posts using post ID, scrape posts by URL, or extract multiple posts from a user’s profile. The API is versatile enough to cover a wide range of scraping needs.
Custom Dataset Solutions
Do you need customized datasets or advanced scraping solutions tailored to your specific requirements? TaskAGI also offers tailor-made dataset creation services and custom scraper development to suit your business needs. Our services are designed to help you gather data that drives growth and adds value to your operations. Contact us to learn more.
Conclusion
TaskAGI’s LinkedIn Scraper API provides a convenient and reliable way to extract data from LinkedIn without dealing with the complexities of direct web scraping. Whether you’re performing competitor analysis, content engagement tracking, or industry trend monitoring, TaskAGI’s solution helps you focus on deriving meaningful insights without worrying about technical barriers or compliance issues.
If you have any questions, leave them in the comments, and if you found this tutorial helpful, consider subscribing for more in-depth data scraping guides and insights!
Leave a Reply