LinkedIn profiles are a treasure trove of information for business networking, market research, recruitment, and much more. Extracting this data manually can be time-consuming, and direct scraping methods often lead to challenges due to LinkedIn’s changing structure and strict policies. Thankfully, TaskAGI’s LinkedIn Scraper API makes it straightforward to gather profile data in a compliant way.
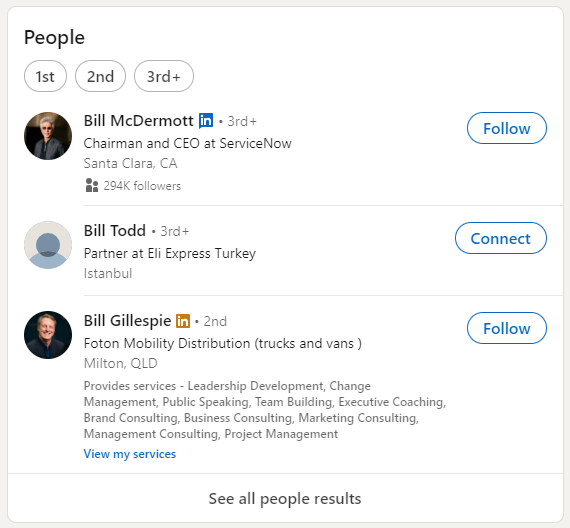
In this guide, we will cover how to scrape LinkedIn profiles using TaskAGI’s API with Python. TaskAGI offers two endpoints, allowing you to either retrieve a profile by username or search for profiles by name.
PS: This post explains how to scrape profile data from LinkedIn. Check this if you want to scrape LinkedIn posts.
Getting Started
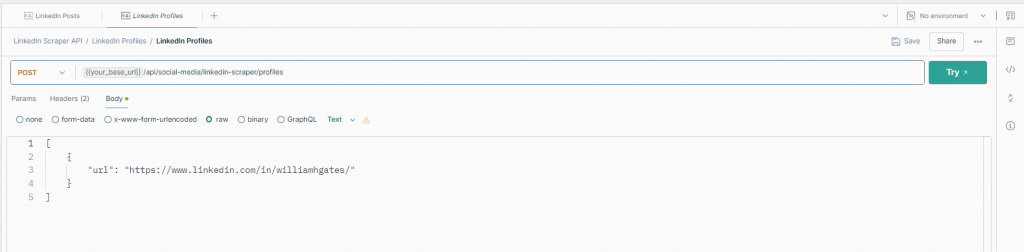
To start, make sure you have access to TaskAGI’s LinkedIn Scraper API. You can find all relevant details about the API at LinkedIn Profile Scraper or through RapidAPI.
Once you have registered and obtained your API key, you are ready to integrate it into your Python script.
Prerequisites
To use the LinkedIn Scraper API, you will need:
- Python 3.6 or higher
- The
requests
library to manage HTTP requests
You can install the requests
library by running the following command:
pip install requests
Endpoints Overview
TaskAGI provides two main endpoints for scraping LinkedIn profiles:
Endpoint | Description |
---|---|
/profiles | Retrieve a LinkedIn profile by username. |
/profiles-by-name | Search for LinkedIn profiles by name. |
Refer to the TaskAGI LinkedIn documentation for detailed information on how to use each endpoint.
Sample Python Script for Scraping LinkedIn Profiles
Below is an example of how to scrape a LinkedIn profile using TaskAGI’s LinkedIn Scraper API:
import requests
import json
# API details
api_url = "https://taskagi.net/api/social-media/linkedin-scraper/profiles"
api_key = "YOUR_API_KEY" # Replace with your actual API key
# Parameters for scraping
payload = {
"username": "williamhgates" # Replace with the target username
}
# Headers with API key for authentication
headers = {
"Authorization": f"Bearer {api_key}",
"Content-Type": "application/json"
}
# Function to scrape LinkedIn profile
def scrape_linkedin_profile():
response = requests.post(api_url, headers=headers, json=payload)
if response.status_code == 200:
data = response.json()
print(json.dumps(data, indent=4)) # Pretty-print the JSON response
else:
print(f"Error: {response.status_code}")
print(response.text)
# Run the function to scrape profile
if __name__ == "__main__":
scrape_linkedin_profile()
Explanation of Script
- API URL & Authentication: The script uses the
/profiles
endpoint, and you need to replaceYOUR_API_KEY
with your TaskAGI API key for authentication. - Payload: The payload requires the LinkedIn username of the profile you wish to scrape. You can change this value to scrape a different profile.
- HTTP Headers: The headers contain the API key, which grants you access to the API.
- Request Execution: The
scrape_linkedin_profile()
function sends a POST request to the API, and if successful, prints the response data in a formatted JSON structure.
Extended Use Cases
Scraping LinkedIn profiles can help you in several scenarios:
- Market Research: Analyze the profiles of industry leaders and gather insights into their professional journey, education, and skills.
- Talent Acquisition: Extract data on potential candidates and build targeted lists for recruitment purposes.
- Lead Generation: Identify key decision-makers and stakeholders in relevant industries for outreach campaigns.
- Networking: Gather information about individuals to facilitate more informed communication in business meetings and events.
- Competitive Analysis: Track career moves and analyze the backgrounds of key players in your industry.
Customizing the Script for Enhanced Functionality
TaskAGI’s LinkedIn Scraper API allows you to gather a range of data points from profiles, including experience, education, posts, and followers. You can further customize the script to extract, store, and analyze these data points.
- Save Data to CSV: Save the profile data to a CSV file for easier access and analysis:
import pandas as pd
# Save scraped profile to CSV
def save_to_csv(data):
df = pd.DataFrame([data])
df.to_csv("linkedin_profile_data.csv", index=False)
print("Data saved to linkedin_profile_data.csv")
- Error Handling: Implement retry logic for better resilience in the face of network issues:
import time
def scrape_with_retries(retries=3):
for attempt in range(retries):
response = requests.post(api_url, headers=headers, json=payload)
if response.status_code == 200:
return response.json()
else:
print(f"Attempt {attempt + 1} failed: {response.status_code}")
time.sleep(2) # Wait before retrying
return None
- Filter Data by Specific Fields: Customize the response data to extract only the fields of interest, such as experience or education.
How does TaskAGI compare?
Many LinkedIn scraping tools in the market, such as PhantomBuster, SalesQL, and TexAu, provide similar capabilities but often come with certain limitations. Here’s how TaskAGI’s LinkedIn Scraper API stands out:
- Wide Range of Data: TaskAGI’s scraper provides comprehensive data including posts, experience, followers, education, and more, which some other tools either limit or require premium plans to access.
- Ease of Integration: Unlike some competitors that require using complex workflows or browser automation, TaskAGI provides simple API endpoints that are easy to integrate with your applications.
- Data Compliance: TaskAGI’s focus on data compliance ensures that scraping remains within the boundaries of LinkedIn’s terms of service, reducing the risk of account suspensions.
- Scalability: TaskAGI’s API is designed to handle larger datasets, making it suitable for enterprise-level use. Many other tools struggle with scalability or impose stricter limits on API usage.
Sample Response
A typical successful response from TaskAGI’s LinkedIn profile scraper API might look like this:
[
{
"id": "williamhgates",
"name": "Bill Gates",
"city": "Seattle, Washington, United States",
"country_code": "US",
"about": "Co-chair of the Bill & Melinda Gates Foundation. Founder of Breakthrough Energy…",
"posts": [
{
"title": "Highlights of my trip to Nigeria and Ethiopia",
"attribution": "By Bill Gates",
"img": "https://media.licdn.com/dms/image/v2/D5612AQF09f3z7Lg5wA/article-cover_image-shrink_600_2000/0/1725593161184",
"link": "https://www.linkedin.com/pulse/highlights-my-trip-nigeria-ethiopia-bill-gates-jqtvc",
"created_at": "2024-09-06T00:00:00.000Z"
}
],
"current_company": {
"link": "https://www.linkedin.com/company/bill-&-melinda-gates-foundation",
"name": "Bill & Melinda Gates Foundation",
"industry": "Philanthropy"
},
"education": [
{
"title": "Harvard University",
"url": "https://www.linkedin.com/school/harvard-university/",
"start_year": "1973",
"end_year": "1975"
}
],
"followers": 35941941,
"connections": 8
}
]
This response includes various profile data points like the name, location, about section, posts, current company, education, followers, and connections, all of which are useful for in-depth analysis and research.
FAQ
1. Can I scrape private LinkedIn profiles?
No, TaskAGI’s LinkedIn Scraper API only allows the scraping of public profile data, ensuring compliance with LinkedIn’s terms of service. Attempting to scrape private profiles can lead to account suspension and legal action.
2. Are there any rate limits on the API?
Yes, there are rate limits to ensure fair use of the API. Please check the API documentation for information on specific rate limits for your plan.
3. What details can I scrape from a profile?
You can extract information such as the user’s name, location, summary, posts, education, current company, and followers, among other data points.
4. How does TaskAGI’s API ensure compliance?
TaskAGI ensures compliance by providing APIs that only allow the scraping of publicly available data, helping users avoid unauthorized access and potential legal issues.
Custom Dataset Solutions
If your business requires more advanced or specialized data solutions, TaskAGI also offers tailored dataset creation services and custom scraper development. Our team can create specific scraping workflows to cater to your unique requirements, providing you with the data that drives insights and growth. Contact us to learn more.
Conclusion
TaskAGI’s LinkedIn Profile Scraper API makes it easy to extract data from LinkedIn profiles without the hassle of complex scraping setups. Whether you need data for market research, lead generation, or talent acquisition, TaskAGI offers a solution that is compliant and reliable, saving you time and effort.
If you have any questions or feedback, leave a comment below. If you found this guide helpful, consider subscribing for more insights on scraping data from social media platforms!
Leave a Reply