LinkedIn is a powerful platform for job seekers, recruiters, and professionals looking to connect. Extracting data on job postings can be immensely valuable for conducting market analysis, gathering hiring trends, or generating targeted leads. Instead of navigating LinkedIn manually or struggling with web scraping complexities, TaskAGI’s LinkedIn Scraper API offers a straightforward solution to scrape job postings in a compliant manner.
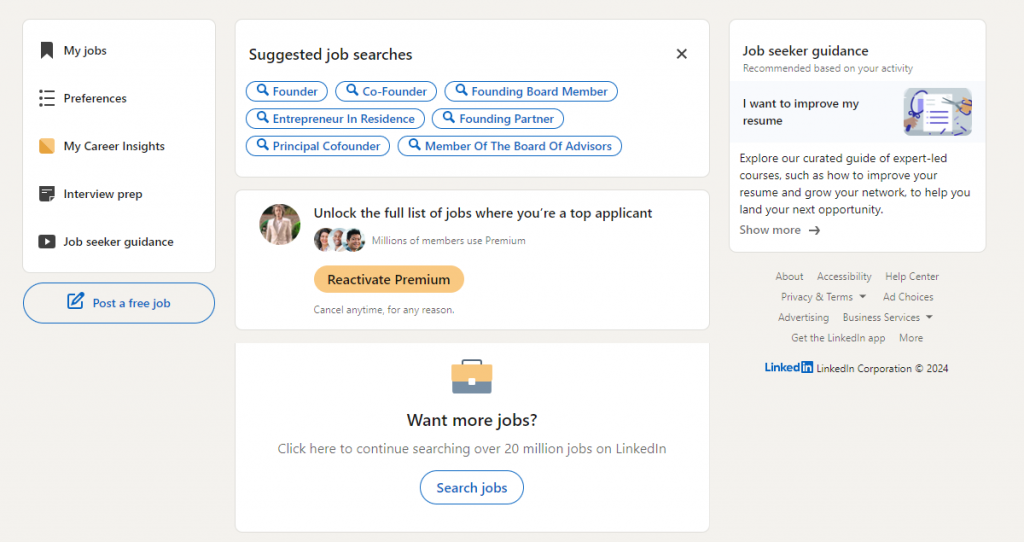
In this guide, we will show you how to scrape LinkedIn job postings using TaskAGI’s API with Python. TaskAGI offers three endpoints for scraping job data: LinkedIn Job by URL, LinkedIn Jobs by Keyword, and LinkedIn Jobs by Company.
Also read: How to Scrape LinkedIn Posts?
Getting Started
To get started, make sure you have access to TaskAGI’s LinkedIn Scraper API. You can find more details at LinkedIn job scraper or through RapidAPI.
Once you have registered and obtained your API key, you are ready to integrate the API into your Python script.
Also read: How to Scrape LinkedIn Profiles?
Prerequisites
To use the LinkedIn Scraper API, you will need:
- Python 3.6 or higher
- The
requests
library for handling HTTP requests
You can install the requests
library by running the following command:
pip install requests
Endpoints Overview
TaskAGI provides three main endpoints for scraping LinkedIn job postings:
Endpoint | Description |
---|---|
/jobs | Retrieve a LinkedIn job by its URL. |
/jobs-by-keyword | Search for LinkedIn jobs by keyword and parameters (e.g., location, job type, experience level). |
/jobs-by-company | Retrieve all job listings from a specific company using the company jobs URL. |
Refer to the TaskAGI LinkedIn documentation for more detailed information about using these endpoints.
Sample Python Script for Scraping LinkedIn Jobs
Below are sample scripts for each endpoint to demonstrate how to scrape LinkedIn job postings.
1. Scrape LinkedIn Job by URL
import requests
import json
# API details
api_url = "https://taskagi.net/api/social-media/linkedin-scraper/jobs"
api_key = "YOUR_API_KEY" # Replace with your actual API key
# Parameters for scraping
payload = {
"url": "https://www.linkedin.com/jobs/view/junior-web-developer-react-js-at-team-remotely-inc-4050444004"
}
# Headers with API key for authentication
headers = {
"Authorization": f"Bearer {api_key}",
"Content-Type": "application/json"
}
# Function to scrape LinkedIn job
def scrape_linkedin_job():
response = requests.post(api_url, headers=headers, json=payload)
if response.status_code == 200:
data = response.json()
print(json.dumps(data, indent=4)) # Pretty-print the JSON response
else:
print(f"Error: {response.status_code}")
print(response.text)
# Run the function to scrape the job
if __name__ == "__main__":
scrape_linkedin_job()
2. Search LinkedIn Jobs by Keyword
# API details
api_url = "https://taskagi.net/api/social-media/linkedin-scraper/jobs-by-keyword"
api_key = "YOUR_API_KEY" # Replace with your actual API key
# Parameters for scraping jobs by keyword
payload = {
"location": "New York",
"keyword": "data analyst",
"country": "US",
"time_range": "Any time",
"job_type": "Part-time",
"experience_level": "Entry level",
"remote": "Remote"
}
# Function to search LinkedIn jobs by keyword
def search_linkedin_jobs():
response = requests.post(api_url, headers=headers, json=payload)
if response.status_code == 200:
data = response.json()
print(json.dumps(data, indent=4)) # Pretty-print the JSON response
else:
print(f"Error: {response.status_code}")
print(response.text)
# Run the function to search for jobs
if __name__ == "__main__":
search_linkedin_jobs()
3. Scrape LinkedIn Jobs by Company
# API details
api_url = "https://taskagi.net/api/social-media/linkedin-scraper/jobs-by-company"
api_key = "YOUR_API_KEY" # Replace with your actual API key
# Parameters for scraping jobs by company
payload = {
"url": "https://www.linkedin.com/jobs/semrush-jobs?f_C=2821922"
}
# Function to scrape jobs by company
def scrape_linkedin_company_jobs():
response = requests.post(api_url, headers=headers, json=payload)
if response.status_code == 200:
data = response.json()
print(json.dumps(data, indent=4)) # Pretty-print the JSON response
else:
print(f"Error: {response.status_code}")
print(response.text)
# Run the function to scrape company jobs
if __name__ == "__main__":
scrape_linkedin_company_jobs()
Explanation of Scripts
- API URL & Authentication: Each script uses a different endpoint (
/jobs
,/jobs-by-keyword
,/jobs-by-company
) to retrieve job data. ReplaceYOUR_API_KEY
with your TaskAGI API key for authentication. - Payload: The payload includes the necessary parameters for scraping. Each endpoint has different requirements, such as job URL, keywords, or company URL.
- HTTP Headers: The headers contain the API key to ensure secure access.
- Request Execution: The functions send a POST request to the API, and if successful, they print the retrieved data in a formatted JSON structure.
Extended Use Cases
Scraping LinkedIn job postings provides significant advantages across multiple scenarios:
- Market Analysis: Extract job data to analyze hiring trends, salary ranges, job types, and skill demands in specific industries.
- Talent Sourcing: Identify companies that are actively hiring and target specific job openings for outreach or recruitment.
- Lead Generation: Gather information about companies hiring for roles relevant to your product, enabling more targeted outreach to key decision-makers.
- Job Aggregation: Create a job aggregator platform that displays the latest job listings based on keywords, location, and company.
Customizing the Scripts for Enhanced Functionality
TaskAGI’s LinkedIn Job Scraper API provides flexibility in extracting various data points such as company information, job summary, and application details. You can further customize the scripts for advanced functionalities.
- Save Job Data to CSV: Save the scraped job data to a CSV file for easier access and analysis:
import pandas as pd
# Save scraped job data to CSV
def save_to_csv(data):
df = pd.DataFrame(data)
df.to_csv("linkedin_job_data.csv", index=False)
print("Data saved to linkedin_job_data.csv")
- Error Handling: Implement retry logic for resilience in case of network issues or rate limits:
import time
def scrape_with_retries(api_url, payload, retries=3):
for attempt in range(retries):
response = requests.post(api_url, headers=headers, json=payload)
if response.status_code == 200:
return response.json()
else:
print(f"Attempt {attempt + 1} failed: {response.status_code}")
time.sleep(2) # Wait before retrying
return None
- Filter Job Data by Specific Fields: You can filter the response to extract only relevant fields such as job title, company name, location, and employment type.
Sample Response
A successful response from TaskAGI’s LinkedIn job scraper API may look like this:
[
{
"input": {
"url": "https://www.linkedin.com/jobs/search/?currentJobId=4032667861&keywords=Member%20Of%20The%20Board%20Of%20Advisors"
},
"url": "https://www.linkedin.com/jobs/view/junior-web-developer-react-js-at-team-remotely-inc-4050444004",
"job_posting_id": "4050444004",
"job_title": "Member of the Board of Advisors",
"company_name": "EPL Group Inc.",
"company_id": "86206867",
"job_location": "United States",
"job_summary": "Sustainly Ventures, an early-stage VC firm focused on ClimateTech , is based in Miami, Florida, and is currently in the process of launching...",
"job_seniority_level": "Executive",
"job_function": "General Business",
"job_employment_type": "Full-time",
"job_industries": "Holding Companies",
"company_url": "https://www.linkedin.com/company/epl-group-inc?trk=public_jobs_topcard-org-name",
"job_posted_time": "1 day ago",
"job_num_applicants": 182,
"apply_link": "https://www.linkedin.com/jobs/view/externalApply/4050444004?url=https%3A%2F%2Fjoin%2Ecom%2Fcompanies%2Feplgroup%2F11052877-member-of-the-board-of-advisors",
"company_logo": "https://media.licdn.com/dms/image/v2/D4E0BAQELLlXmNr4Dcw/company-logo_100_100/0/1691931867843/epl_group_inc_logo",
"job_posted_date": "2024-10-13T20:49:08.042Z"
}
]
This response contains detailed information such as job title, company name, job location, job summary, employment type, and more, which can be used for analysis or aggregation.
FAQ
1. Can I scrape private LinkedIn job postings?
No, TaskAGI’s LinkedIn Scraper API only allows scraping of publicly available job postings. Scraping private or restricted content could lead to account suspension and legal issues.
3. What job details can I extract?
You can extract various job details, including job title, company, job summary, location, employment type, seniority level, application link, and the number of applicants.
4. Can I use TaskAGI’s scraper for commercial purposes?
Yes, provided you comply with LinkedIn’s terms of service and TaskAGI’s usage policies, you can use the scraped data for commercial purposes.
Custom Dataset Solutions
Do you need custom datasets or advanced scraping solutions tailored to your specific requirements? TaskAGI offers tailored dataset creation services and custom scraper development to suit your business needs. Contact us to learn more.
Conclusion
TaskAGI’s LinkedIn Job Scraper API makes extracting job data from LinkedIn easy and effective. Whether you’re interested in conducting market research, lead generation, or aggregating job postings, TaskAGI provides a reliable and compliant solution that saves time and effort.
If you have any questions or feedback, feel free to leave a comment below. If you found this guide helpful, consider subscribing for more insights on scraping data from social media platforms!
Leave a Reply