LinkedIn company pages are valuable resources for gathering information about businesses, such as their size, locations, specialties, and recent updates. Extracting this data can be highly beneficial for market research, competitor analysis, or generating targeted business leads. Instead of manually navigating LinkedIn or dealing with the complexities of scraping, TaskAGI’s LinkedIn Scraper API offers an easy and compliant solution.
In this guide, we’ll show you how to scrape LinkedIn company information using TaskAGI’s API with Python. The endpoint used for this purpose is /api/social-media/linkedin-scraper/companies
, which takes the LinkedIn URL of the company page as an argument.
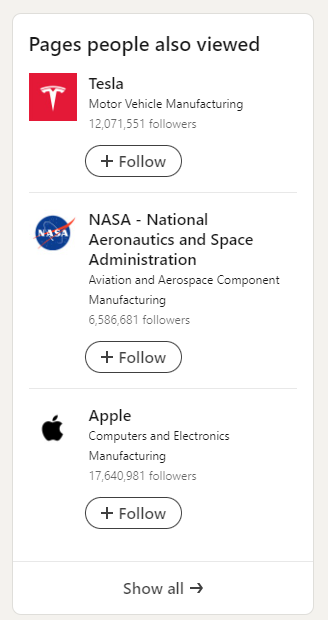
Getting Started
To get started, make sure you have access to TaskAGI’s LinkedIn Scraper API. You can find more details at TaskAGI’s official website or through RapidAPI.
Once you have registered and obtained your API key, you can integrate the API into your Python script.
Related: How to Scrape LinkedIn Job Postings Using Python?
Prerequisites
To use the LinkedIn Scraper API, you will need:
- Python 3.6 or higher
- The
requests
library to handle HTTP requests
You can install the requests
library by running the following command:
pip install requests
Endpoint Overview
TaskAGI provides a single endpoint for scraping LinkedIn company pages:
Endpoint | Description |
---|---|
/companies | Retrieve data from a LinkedIn company page by URL. |
Sample Python Script for Scraping LinkedIn Company Data
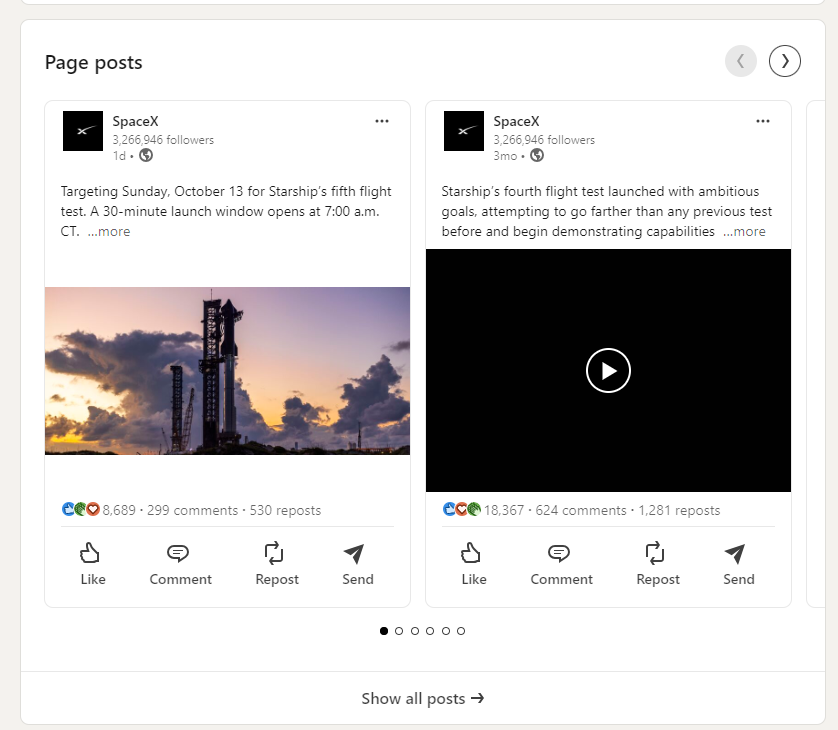
Below is a sample script to demonstrate how to scrape LinkedIn company information using the TaskAGI API:
import requests
import json
# API details
api_url = "https://taskagi.net/api/social-media/linkedin-scraper/companies"
api_key = "YOUR_API_KEY" # Replace with your actual API key
# Parameters for scraping
payload = {
"url": "https://www.linkedin.com/company/ibm" # Replace with the LinkedIn company URL you want to scrape
}
# Headers with API key for authentication
headers = {
"Authorization": f"Bearer {api_key}",
"Content-Type": "application/json"
}
# Function to scrape LinkedIn company data
def scrape_linkedin_company():
response = requests.post(api_url, headers=headers, json=payload)
if response.status_code == 200:
data = response.json()
print(json.dumps(data, indent=4)) # Pretty-print the JSON response
else:
print(f"Error: {response.status_code}")
print(response.text)
# Run the function to scrape the company data
if __name__ == "__main__":
scrape_linkedin_company()
Explanation of the Script
Also read: How to Scrape LinkedIn Profiles Using Python?
- API URL & Authentication: The script uses the
/companies
endpoint. ReplaceYOUR_API_KEY
with your TaskAGI API key for authentication. - Payload: The payload includes the LinkedIn company URL that you wish to scrape.
- HTTP Headers: The headers contain the API key to ensure secure access to the API.
- Request Execution: The function sends a POST request to the API, and if successful, prints the retrieved company data in a formatted JSON structure.
Extended Use Cases
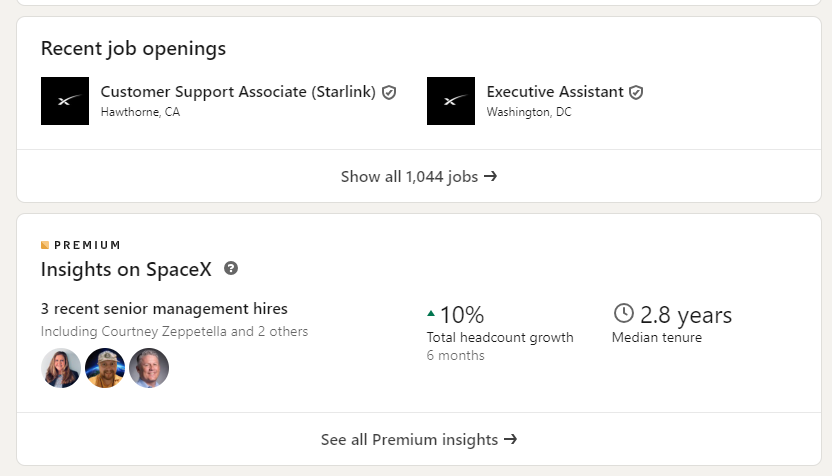
Scraping LinkedIn company pages can be useful for multiple scenarios:
- Market Research: Extract company information such as headquarters, locations, and industries to analyze market trends and identify business opportunities.
- Competitor Analysis: Gather data on competitors, including company size, specialties, and recent activities to gain insights into their strategies.
- Sales Intelligence: Identify potential clients or partners by gathering detailed information about companies, including their specialties and employee distribution.
- Lead Generation: Use the follower count and company updates to identify growing companies that may be interested in your services.
Customizing the Script for Enhanced Functionality
Also read: How to Scrape LinkedIn Posts Using Python?
TaskAGI’s LinkedIn Company Scraper API provides a wealth of information that can be further customized to meet your needs.
- Save Company Data to CSV: Save the scraped company data to a CSV file for easier analysis and record-keeping:
import pandas as pd
# Save scraped company data to CSV
def save_to_csv(data):
df = pd.DataFrame([data])
df.to_csv("linkedin_company_data.csv", index=False)
print("Data saved to linkedin_company_data.csv")
- Error Handling: Implement retry logic for resilience in case of network issues or rate limits:
import time
def scrape_with_retries(api_url, payload, retries=3):
for attempt in range(retries):
response = requests.post(api_url, headers=headers, json=payload)
if response.status_code == 200:
return response.json()
else:
print(f"Attempt {attempt + 1} failed: {response.status_code}")
time.sleep(2) # Wait before retrying
return None
- Extract Specific Data Points: You can filter the response to extract specific fields of interest, such as employee count, headquarters, or company description.
Sample Response
A successful response from TaskAGI’s LinkedIn company scraper API may look like this:
[
{
"input": {
"url": "https://il.linkedin.com/company/ibm"
},
"id": "ibm",
"name": "IBM",
"country_code": "US,ZA,UY,TH,ID,AU,CZ,SG,IT,DE,MY,FI,CO,RU,AE,FR,SK,KW,IN,BR,GR,MX,ES,AR,RO,EG",
"locations": [
"International Business Machines Corp. New Orchard Road Armonk, New York, NY 10504, US",
"590 Madison Ave New York, NY 10022, US",
"90 Grayston Dr Sandton, Gauteng 2196, ZA",
"Plaza Independencia 721 Montevideo, 11000, UY"
],
"followers": 17226208,
"employees_in_linkedin": 314873,
"about": "At IBM, we do more than work. We create. We create as technologists, developers, and engineers. We create with our partners. We create with our competitors. If you're searching for ways to make the world work better through technology and infrastructure, software and consulting, then we want to work with you. We're here to help every creator turn their \"what if\" into what is. Let's create something that will change everything.",
"specialties": "Cloud, Mobile, Cognitive, Security, Research, Watson, Analytics, Consulting, Commerce, Experience Design, Internet of Things, Technology support, Industry solutions, Systems services, Resiliency services, Financing, and IT infrastructure",
"company_size": "10,001+ employees",
"organization_type": "Public Company",
"industries": "IT Services and IT Consulting",
"website": "https://www.ibm.com/",
"company_id": "1009",
"headquarters": "Armonk, New York, NY"
}
]
This response includes detailed company information such as name, locations, specialties, employee count, headquarters, and more, which can be used for further analysis or decision-making.
FAQ
1. Can I scrape data from any LinkedIn company page?
Yes, TaskAGI’s LinkedIn Company Scraper API allows scraping from any publicly accessible LinkedIn company page. Note that pages with restricted or private settings may not be accessible.
2. What kind of company details can I extract?
The API allows you to extract various details, including company name, locations, specialties, followers, employee count, industries, company size, headquarters, and more.
3. How often can I scrape data using the API?
The frequency of scraping is determined by the rate limits on your API plan. Ensure you comply with LinkedIn’s terms of service when deciding the frequency of your data extraction. For more details on rate limits, refer to the API documentation.
4. Can I use the scraped company data for commercial purposes?
Yes, you can use the data for commercial purposes, as long as it complies with LinkedIn’s terms of service and TaskAGI’s usage policies.
5. Can I automate the scraping process for multiple companies?
Absolutely! You can modify the script to loop through a list of LinkedIn company URLs and automate the scraping process for multiple companies. Just ensure you manage rate limits and avoid any actions that could violate LinkedIn’s terms of service.
Custom Dataset Solutions
If your business needs custom datasets or advanced scraping solutions tailored to specific requirements, TaskAGI also offers custom scraper development and tailored dataset creation services. Contact us to learn more about how we can help.
Conclusion
TaskAGI’s LinkedIn Company Scraper API provides an efficient and compliant way to extract valuable data from LinkedIn company pages. Whether you’re conducting competitor analysis, market research, or generating sales leads, this API provides the tools you need to make informed decisions.
If you have any questions or feedback, feel free to leave a comment below. If you found this guide helpful, consider subscribing for more insights on scraping data from social media platforms!
Leave a Reply