Airbnb offers a vast amount of data on properties, from amenities to customer reviews, making it an invaluable source of information for real estate research, market analysis, and vacation planning. Instead of manually browsing through listings, TaskAGI’s Airbnb Scraper API allows you to extract relevant data quickly and efficiently.
In this guide, we’ll show you how to scrape Airbnb property details and reviews using TaskAGI’s API with Python. There are two endpoints available: one for collecting data by URL and another for collecting data by location.
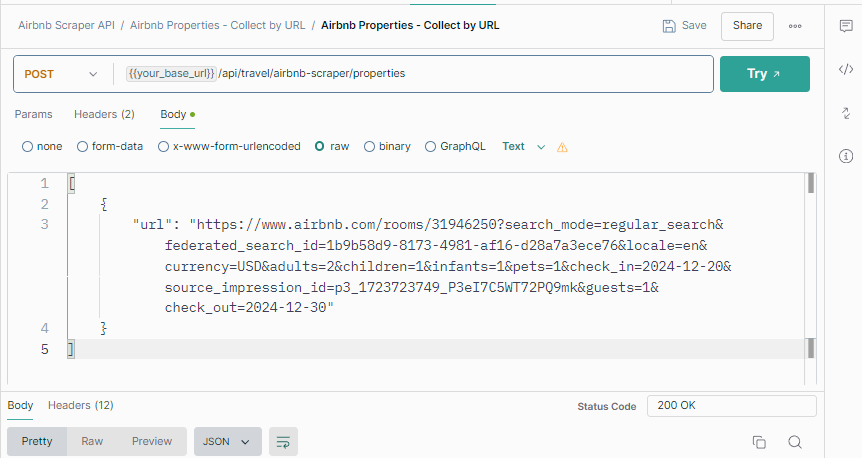
Getting Started
To get started, ensure you have access to TaskAGI’s Airbnb Scraper API. More details can be found at AirBnB Scraper or through RapidAPI.
Once you have registered and obtained your API key, you can integrate the API into your Python script.
Prerequisites
To use the Airbnb Scraper API, you will need:
- Python 3.6 or higher
- The
requests
library to handle HTTP requests
You can install the requests
library by running the following command:
pip install requests
Endpoint Overview
TaskAGI provides two endpoints for scraping Airbnb properties:
Endpoint | Description |
---|---|
/properties | Retrieve property data by Airbnb URL. |
/properties-by-location | Retrieve property data by specifying a location. |
Refer to the TaskAGI Airbnb Scraper documentation for more detailed information.
Sample Python Script for Scraping Airbnb Property Data
Below is a sample script to demonstrate how to scrape Airbnb property information using the TaskAGI API:
import requests
import json
# API details
api_url = "https://taskagi.net/api/travel/airbnb-scraper/properties"
api_key = "YOUR_API_KEY" # Replace with your actual API key
# Parameters for scraping
payload = {
"url": "https://www.airbnb.com/rooms/31946250?check_in=2024-12-20&check_out=2024-12-30&guests=1" # Replace with the Airbnb property URL you want to scrape
}
# Headers with API key for authentication
headers = {
"Authorization": f"Bearer {api_key}",
"Content-Type": "application/json"
}
# Function to scrape Airbnb property data
def scrape_airbnb_property():
response = requests.post(api_url, headers=headers, json=payload)
if response.status_code == 200:
data = response.json()
print(json.dumps(data, indent=4)) # Pretty-print the JSON response
else:
print(f"Error: {response.status_code}")
print(response.text)
# Run the function to scrape the property data
if __name__ == "__main__":
scrape_airbnb_property()
Explanation of the Script
- API URL & Authentication: The script uses the
/properties
endpoint. ReplaceYOUR_API_KEY
with your TaskAGI API key for authentication. - Payload: The payload includes the Airbnb property URL that you wish to scrape.
- HTTP Headers: The headers contain the API key to ensure secure access to the API.
- Request Execution: The function sends a POST request to the API, and if successful, prints the retrieved property data in a formatted JSON structure.
Use Cases for Scraping Airbnb Data
Scraping Airbnb properties can be useful for a variety of scenarios:
- Market Research: Extract property details such as pricing, ratings, and amenities to analyze trends in vacation rentals or compare properties across different regions.
- Real Estate Analysis: Gather data on properties to evaluate investment opportunities in popular vacation destinations.
- Competitor Monitoring: Understand competitor pricing, reviews, and property availability to adjust your own rental strategy.
- Travel Planning: Extract details of properties to find the best accommodation that meets your specific needs.
Customizing the Script for Enhanced Functionality
TaskAGI’s Airbnb Scraper API provides a wealth of information that can be further customized to meet your needs.
- Collect Data by Location: To collect Airbnb properties by location, use the
/properties-by-location
endpoint. You can modify the script to scrape multiple properties within a specific geographic area:
api_url = "https://taskagi.net/api/travel/airbnb-scraper/properties-by-location"
payload = {
"location": "Europe",
"num_of_infants": ""
}
def scrape_airbnb_by_location():
response = requests.post(api_url, headers=headers, json=payload)
if response.status_code == 200:
data = response.json()
print(json.dumps(data, indent=4))
else:
print(f"Error: {response.status_code}")
print(response.text)
if __name__ == "__main__":
scrape_airbnb_by_location()
- Error Handling: Add error handling and retry logic to manage rate limits and handle network issues:
import time
def scrape_with_retries(api_url, payload, retries=3):
for attempt in range(retries):
response = requests.post(api_url, headers=headers, json=payload)
if response.status_code == 200:
return response.json()
else:
print(f"Attempt {attempt + 1} failed: {response.status_code}")
time.sleep(2) # Wait before retrying
return None
- Save Property Data to CSV: Save the scraped property data to a CSV file for easier analysis and record-keeping:
import pandas as pd
# Save scraped property data to CSV
def save_to_csv(data):
df = pd.DataFrame([data])
df.to_csv("airbnb_property_data.csv", index=False)
print("Data saved to airbnb_property_data.csv")
Sample Response
A successful response from TaskAGI’s Airbnb Scraper API may look like this:
[
{
"input": {
"url": "https://www.airbnb.com/rooms/31946250"
},
"name": "Villa in Korinos · ★4.76 · 5 bedrooms · 7 beds · 3.5 baths",
"image": "https://a0.muscache.com/pictures/f979d519-40b2-4c18-9e74-049e84204314.jpg",
"description": "The villa consists of 5 bedrooms with 3 double beds...",
"category": "Stays",
"availability": "false",
"reviews": [
"Very nice area, great hostess, clean and well equipped house...",
"Nice, clean, perfect for family vacation.",
"despina was very friendly, which unfortunately went differently..."
],
"ratings": 4.76,
"seller_info": {
"url": "https://www.airbnb.com/users/show/43665162",
"seller_id": "43665162"
},
"breadcrumbs": "Korinos, Greece",
"location": "Korinos, Greece",
"lat": 40.31294,
"long": 22.61731,
"guests": 13,
"pets_allowed": true,
"description_items": [
"Entire villa",
"7 beds",
"3.5 baths"
]
}
]
This response includes detailed property information such as name, location, reviews, ratings, availability, and more, which can be used for further analysis or decision-making.
FAQ
1. Can I scrape data from any Airbnb property?
Yes, TaskAGI’s Airbnb Scraper API allows scraping from any publicly accessible Airbnb property page. Note that some listings with restricted settings may not be accessible.
2. What kind of property details can I extract?
The API allows you to extract various details, including property name, description, availability, reviews, ratings, amenities, and more.
3. How often can I scrape data using the API?
The frequency of scraping is determined by the rate limits on your API plan. Ensure you comply with Airbnb’s terms of service when deciding the frequency of your data extraction.
4. Can I automate the scraping process for multiple properties?
Absolutely! You can modify the script to loop through a list of Airbnb URLs or use the location-based endpoint to scrape multiple properties. Just ensure you manage rate limits and avoid actions that could violate Airbnb’s terms of service.
5. Can I use the scraped property data for commercial purposes?
Yes, you can use the data for commercial purposes, as long as it complies with Airbnb’s terms of service and TaskAGI’s policies. Always review Airbnb’s guidelines to ensure compliance with their data usage policies.
6. What if I encounter rate limiting?
Rate limiting is a restriction on the number of API calls you can make within a specific time period. If you encounter rate limiting, try reducing the frequency of your API requests or consider upgrading your subscription plan for higher limits.
7. How do I handle blocked requests?
If you encounter blocked requests, it could be due to the API rate limits, server issues, or restrictions from Airbnb. To manage this, add a retry mechanism in your script and ensure that the requests are spaced out appropriately to avoid detection or throttling.
8. Can I filter properties by amenities or other features?
Currently, the API allows you to scrape all publicly available property data. Once the data is retrieved, you can apply filters based on the amenities or features that are relevant to your use case.
Leave a Reply